operators
// relational operator
// >, >=, <, <=, <=>
// logical Operators
// negation: !
// &&: logical and
// ||: logical or
// modulus operator, proves possiblity of dividing
int num10 = 3;
if (num10 % 2 == 0) {
cout << num10 << " is an even number.\n";
} else {
cout << num10 << " is an odd number.\n";
}
conditional operator
//example 1
int a = 1;
int b = 2;
int result = (a > b) ? a : b; //if a greater than b, result is a otherwise its b
//example 2
int score = 8;
cout << ((score > 10) ? "good" : "bad") << endl;
// example 3
num1 = 1;
num2 = 3;
if (num1 != num2){
cout << "largest:" << ((num1 > num2) ? num1 : num2) << endl;
cout << "smallest:" << ((num1 < num2) ? num1 : num2) << endl;
} else {
cout << "the numbers are the same" << endl;
}
compound operators
sum += value; //short
sum = sum + value; //long
foo = bar++; //short
temp = bar; //long
bar += 1;
foo = temp;
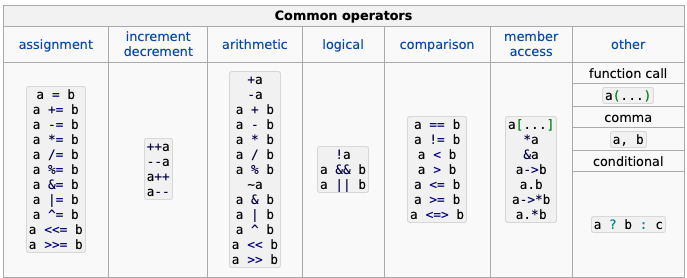