Polymorphism
→ fundamental to Object-Oriented Programming
Polymorphism: Run-time / late binding / dynamic binding (normally before before compiling)
→ Runtime polymorphism: being able to assign different meanings to the same function at run-time
→ Allows to programm more abstractly
→ Polymorphism is achieved via
- inheritance
- base class pointers or references
- virtual functions
Static Binding
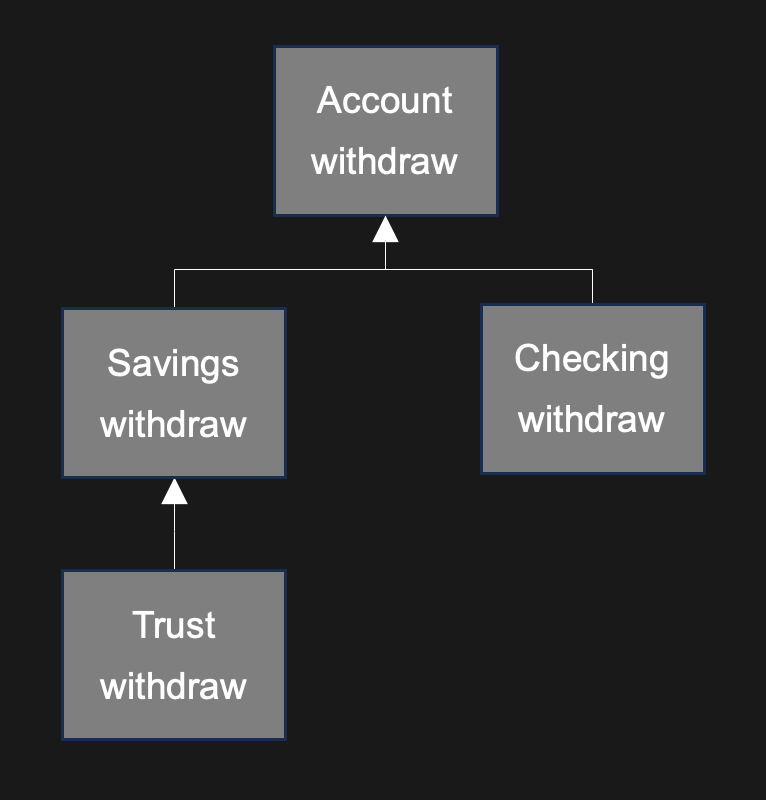
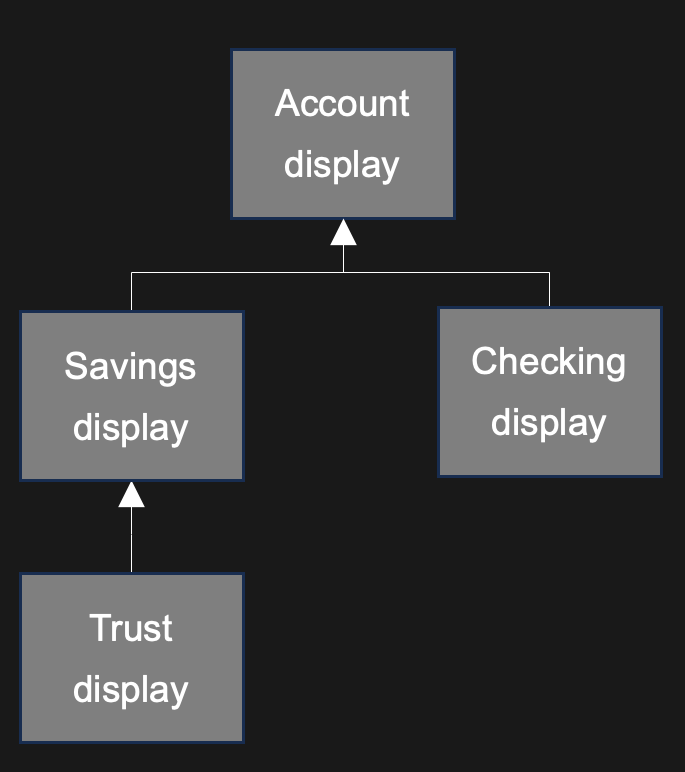
void display_account(const Account &acc) {
acc.display();
// will always use Account::display
}
Account a;
display_account(a);
Savings b;
display_account(b);
Checking c;
display_account(c);
Trust d;
display_account(d);
Dynamic binding (Runtime Polymorphism)
Account a;
a.withdraw(1000); // Account::withdraw()
Savings b;
b.withdraw(1000); // Savings::withdraw()
Checking c;
c.withdraw(1000); // Checking::withdraw()
Trust d;
d.withdraw(1000); // Trust::withdraw()
Account *p = new Trust();
P->withdraw(1000); // Trust::withdraw()
// withdraw method is virtual in Account
void display_account(const Account &acc)
{
acc.display();
// will always call the display method
// depending on the object's type
// at RUN-TIME
}
Account a;
display_account(a);
Savings b;
display_account(b);
Checking c;
display_account(c);
Trust d;
display_account(d);
// example
using Base class pointer
Account *p1 = new Account();
Account *p2 = new Savings();
Account *p3 = new Checking();
Account *p4 = new Trust();
p1->withdraw(1000); // Account::withdraw
p2->withdraw(1000); // Savings::withdraw
p3->withdraw(1000); // Checking::withdraw
p4->withdraw(1000); // Trust::withdraw
// delete the pointers
// usecase
Account *p1 = new Account();
Account *p2 = new Savings();
Account *p3 = new Checking();
Account *p4 = new Trust();
// for array
Account *array [] = {p1, p2, p3, p4};
for (auto i=0; i<4; ++i)
array[i]->withdraw(1000);
// for vector
std::vector<Account *> accounts{p1,p2,p3,p4};
for (auto acc_ptr:accounts)
acc_ptr->withdraw(1000);